I recently bought a new iPad to help me be more productive at work. It didn't take long for me to start wondering if you can use an iPad for programming or SSH access.
And the answers is yes, you can! There's a fair range of IDE and compiler apps for the iPad. Python seems to be one of the more popular languages amongst app makers although you'll still find apps for other languages such as Objective-C and C#.
I ended up picking Pythonista. Pythonista goes a step further than just providing an IDE for writing Python code. It also provides a set of python modules that allows you to interact with the operating system and features of the device. You can access the keychain, trigger notifications, build share extensions, and even build simple user interfaces. This suits me quite well as my goal isn't to try and program on an iPad the same way I would on my laptop and desktop. When I'm programming on the iPad, I'm more interested in seeing if I can build any helpful utilities or automations that can give me a productivity boost.
There are also plenty of SSH apps to choose from – the challenge is picking which one. I ended up settling on Blink as it was fairly inexpensive compared to some of the other options and it allows me to do agent forwarding and a few other bits that competitor apps didn't support.
Python Programming with Pythonista
Pythonista will let you perform most programming tasks, and supports both Python 2.7 and 3.6. I'm mostly using Pythonista for building small automations that will be useful to invoke on the iPad.
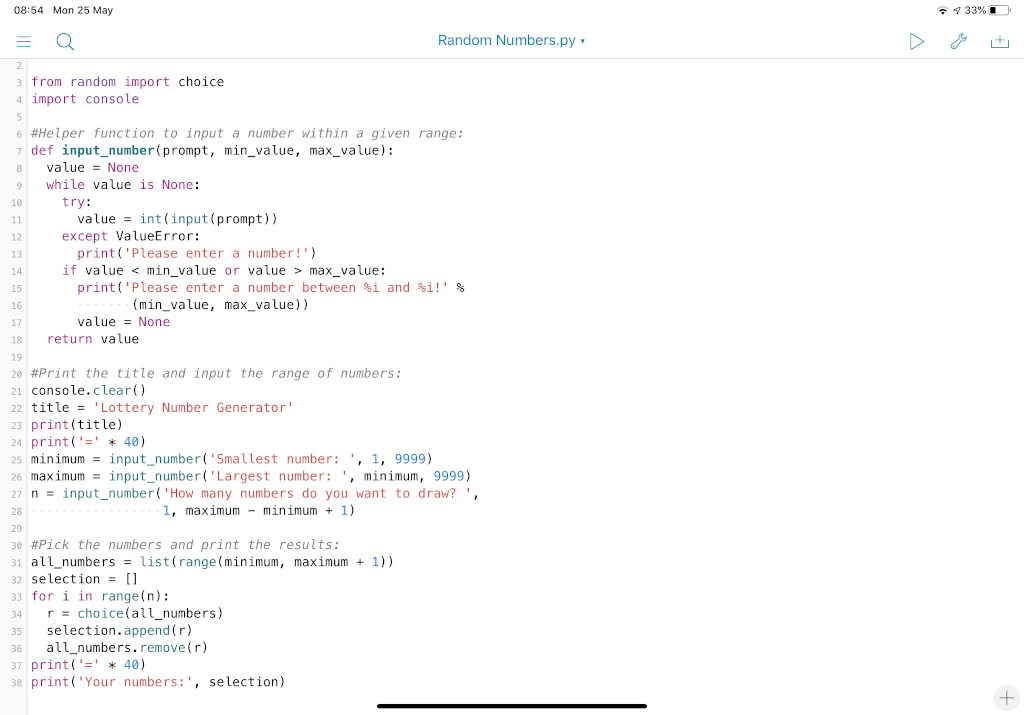
The app comes bundled with a variety of example scripts that show how to use the various Pythonista python modules.
As you will see with some of the example, you can build scripts that require and use a user interface. Pythonista provides a drag-and-drop environment within the app where GUI files can be created and edited
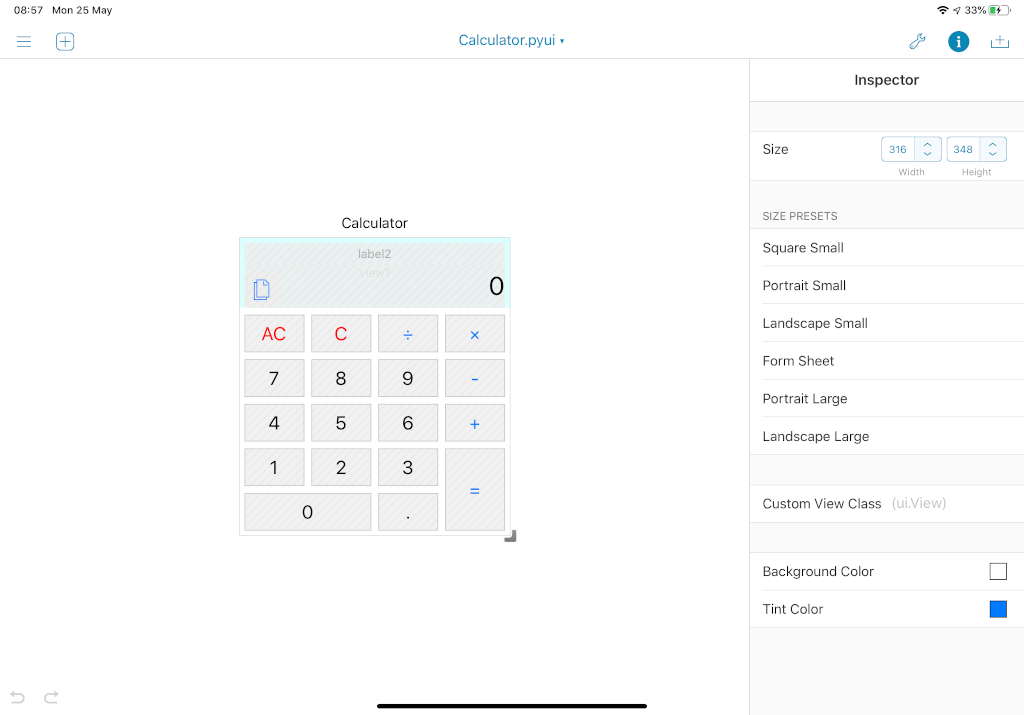
Programming on the iPad can't compete with a fully-fledged desktop programming environment, although I'm impressed with what Pythonista can actually allow you to achieve.
If you're planning on using Pythonista, here are a few things that you may want to consider:
Get StaSh - a Bash-like shell for Pythonista
One of the first things to do with Pythonista is to get and install StaSh. This will provide you with a shell environment where you can easily execute any command you need like pip install
.
StaSh can be installed by using Pythonista to execute this one-liner:
import requests as r; exec(r.get('https://bit.ly/get-stash').content)
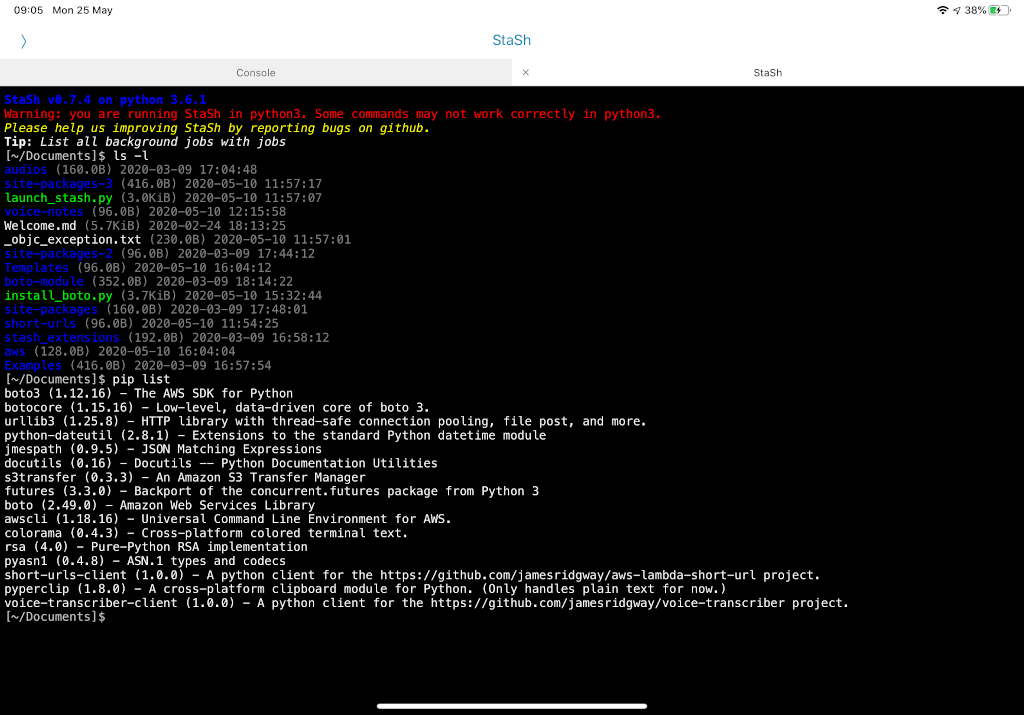
Use the Cloud
Regardless of what app you use for programming, an iPad just doesn't provide a full terminal or UNIX style environment. Some apps for the iPad can get you part of the way there, but there is no substitute for a normal desktop environment.
I work a fair bit in the cloud and one of the first things I built with Pythonista is a basic script that allows me to start/stop/resize an EC2 instance in my AWS account. Whenever I need access I use Pythonista to start it up, and Blink to SSH onto the machine.
The script for doing this is pretty simple:
import keychain
import console
import boto.ec2
import time
aws_access_key_id = keychain.get_password("aws_credentials", "default")
aws_secret_access_key = keychain.get_password("aws_credentials", aws_access_key_id)
conn = boto.ec2.connect_to_region("eu-west-1", aws_access_key_id=aws_access_key_id,aws_secret_access_key=aws_secret_access_key)
def get_instance():
return conn.get_all_reservations(filters={"tag:Name": "Core"})[0].instances[0]
def info(instance):
print("Instance Details")
print("----------------")
print("Instance ID: ", instance.id)
print("Instance Type: ", instance.instance_type)
print("Public IP: ", instance.ip_address)
print("State: ", instance.state)
print()
def stop():
instance = get_instance()
if instance.state != "stopped":
conn.stop_instances(instance_ids=[instance.id])
while instance.state != "stopped":
print("Instance currently: {0}, stopping instance...".format(instance.state))
time.sleep(10)
instance = get_instance()
print("Instance stopped!")
def start():
instance = get_instance()
if instance.state != "running":
conn.start_instances(instance_ids=[instance.id])
while instance.state != "running":
print("Instance currently: {0}, starting instance...".format(instance.state))
time.sleep(10)
instance = get_instance()
print("Instance started!")
print()
info(get_instance())
def resize(size):
stop()
print("Resizing instance to: ", size)
instance = get_instance()
conn.modify_instance_attribute(instance_id=instance.id, attribute='instanceType', value=size)
instance = get_instance()
info(instance)
start()
core_instance = get_instance()
info(core_instance)
print("You can issue the commands: start(), stop() or resize('instance.type')")
print()
As you can see, the script is predominantly just a set of functions. This is because when I run the script in Pythonista, I will get access to a REPL shell once the script has run, where I can execute any method that has been loaded into the environment.
Once I've run the script all I have to do is type start()
into the REPL shell to start it up, and stop()
to stop the instance when I'm done.
This script is also a good example of how I'm able to use the keychain
API that Pythonista provides to be able to store and retrieve my credentials from the operating system keychain.
Beware of limitations/drawbacks
I've only come across a few things to be aware of over the last couple of months, neither of which has caused a major issue for me:
No Native Extensions
Pythonista doesn't support python modules that rely on native compilation or any native dependencies. I'm yet to encounter this as an issue, but it's worth bearing in mind.
Using Boto for AWS with Pythonista
For some reason I've never been able to pip install
boto using StaSh. I've not dug deeper into the reasons why as I can across the following Gist that can be used to install boto:
SSH with Blink
There are no shortages of SSH apps for the iPad. I looked at Blink, Prompt 2 and Terminus.
Terminus is easily the most comprehensive, but it's also the most expensive as it's priced at a monthly subscription that starts from around $8/month. I simply can't see myself using SSH on an iPad enough to justify a price like that.
These days I'm very reliant on being able to use agent forwarding when I SSH into machines. Both Blink and Prompt 2 support SSH agents. After some umming-and-erring I settled on Blink. At the time it seemed to be more actively developed, and it's open source so feature contributions don't have to come directly from the developers, they can come from the community.
Blink isn't a complicated app and it's very straightforward to use. By typing config
you can get to the settings section of the app where you can config:
- Keys
Bring your own, or create a new one - Hosts
Configure which hosts you want to SSH to. All the usual stuff that you'd put in an SSH config file. plus support for Mosh. - Keyboard Settings
Configure various keyboard settings, including on-screen smart keys that can provide soft keys for physical keys that your keyboard may be lacking (e.g. function and escape keys).
config
commandSSH Agent Forwarding
SSH agent forwarding is a little clunky, as you have to run ssh-agent
yourself:
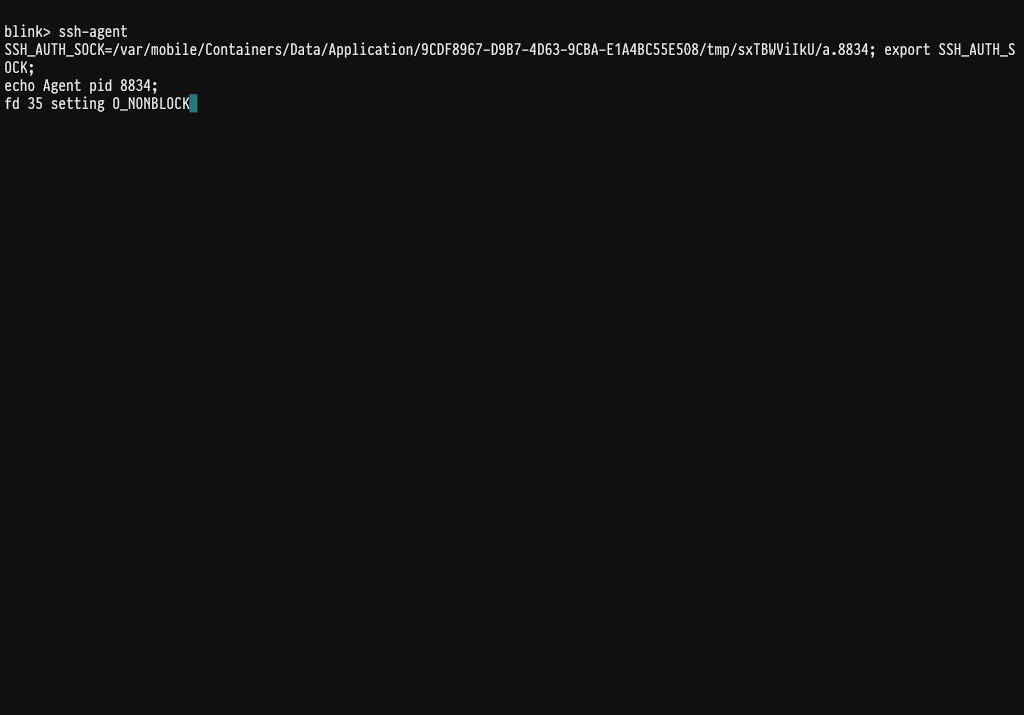
ssh-agent
in a separate tab.Once the agent is running in a tab, it's then just a case of hitting cmd + T
to open a new tab and SSH to the machine of your choosing. Provided you keep the ssh-agent
running in the other tab, SSH agent forward will work for anything that you SSH to.
Conclusion
There are plenty of apps out there for either programming or SSH on the iPad, and they're pretty good when you consider that an iPad isn't designed to be a development environment.
On a day to day basis I get more use out of being able to SSH from the iPad rather than programming. Especially when a monitoring alert may trigger out of hours and the iPad is closer to hand than the laptop. After all, once I'm SSHed into a machine there is very little that I can do on a desktop that cannot be achieved on an iPad SSH session.
For me at least, I can't see the iPad replacing a typical desktop based development environment. The iPad is a very locked down ecosystem, and what I've found of programming on the iPad so far is that there are limitations. But my primary goal for dabbling with programming on the iPad has been to see if I can write a few useful scripts for the iPad that will help boost my productivity.
Over the next few blog posts I'll be sharing these scripts.